第十四章-String类-1
栏目分类:java教程 发布日期:2019-09-21 浏览次数:次
第十四章-String类-1
在Java中字符串被当作对象处理,使用String类来创建、操作字符串对象。String类在lang包中,这里我们不需要进行导包。(lang包是Java的核心包,JVM默认导入而不需要我们再进行显示导入)
这里讲解一下Java中最常用的两种创建数组的方法,方法1使用字符串常量来创建字符串变量。方法2则是使用字符串数组来创建字符串变量。使用字符数组来创建字符串变量还可以将数组中一部分元素提取出来创建字符串变量。具体实现见例程中方法2-2,其操作的元素也是只包括起始范围的值,不包括结束范围的值。字符串必须包含子啊双引号里,声明过后必须初始化才能使用。
public class StringDemo{
public static void main(String[] args){
String one;
one="Hello";
System.out.println(one);//方法1
char two1[]= {'h','e','l','l','o'};
String three1=new String(two1);
System.out.println(three1);//方法2-1
char two2[]= {'h','e','l','l','o'};
String three2=new String(two2,0,2);
System.out.println(three2);//方法2-2
}
}
了解了字符串的声明与创建后我们来学习一下字符串的基本操作,连接、获取字符串长度、查找、获取指定索引位置字符、获取子字符串、去除空格、分割、替换……两个字符串使用“+”运算符可以进行连接,拼合成一个新的字符串。Java中一个字符串是不能分开两行写的,如果字符串过长要分两行写的话也需要使用“+”运算符进行连接,否则会报错。
public class StringDemo{
public static void main(String[] args){
String one,two,three,four;
one="Hello,";
two="world!";
three=one+""+two;
System.out.println(one);
System.out.println(two);
System.out.println(three);
four="Java is "+
"gret";
System.out.println(four);
System.out.println("Java is "+
"gret!");
}
}
除了字符串之间相连接外,还可以与其它基本数据类型进行连接(其它数据类型数据会被转换成字符串,“+”运算符只要有一个操作数是字符串另一个操作数就会被编译器转换成字符串)。
public class StringDemo{
public static void main(String[] args){
int one=2;
float two=2.2F;
String three="版本:";
three=three+one;
System.out.println(three);
System.out.println(three+one+two);
System.out.println(three+(one+two));
}
}
获取字符串长度使用length()方法
public class StringDemo{
public static void main(String[] args){
String one="Hello,world!";
String two="Hello,java!";
int size=one.length();
System.out.println(size);
System.out.println(two.length());
}
}
获取指定索引位置字符使用charAt()方法
public class StringDemo{
public static void main(String[] args){
String one="Hello,world!";
char two=one.charAt(2);
System.out.println(two);
System.out.println(one.charAt(6));
}
}
字符串查找String类提供了indexOf()、lastIndexOf()两种方法。indexOf返回的是被搜素字符(字符串)首次出现位置,lastIndexOf()则是返回被搜素字符(字符串)最后一次出现位置。若没有检索到则会返回-1。
public class StringDemo{
public static void main(String[] args){
String one="Hello,world!";
System.out.println(one.indexOf("w"));
System.out.println(one.indexOf("a"));
System.out.println(one.lastIndexOf("l"));
System.out.println(one.lastIndexOf("a"));
}
}
获取子字符串使用substring()方法,可利用下标对字符串进行截取操作。有两种不同的方法重载。
public class StringDemo{
public static void main(String[] args){
String one="Hello,world!";
System.out.println(one.substring(2));//方法1-从指定下标处开始截取
System.out.println(one.substring(2,6));//方法2-截取指定范围内元素
}
}
去除空格使用trim()方法。trim()方法去除的是首尾空格,这种空格一般无意义而且会影响字符串操作、占用空间。
public class StringDemo{
public static void main(String[] args){
String one=" Hello world ";
String two;
System.out.println(one.length());
System.out.println(one);
System.out.println(one.trim().length());
two=one.trim();
System.out.println(two);
System.out.println(two.length());
}
}
字符串替换使用replace()方法。
public class StringDemo{
public static void main(String[] args){
String one1="Hello,world!";
String one2="Hello,world!";
String one3="Hello,world!";
System.out.println(one1.replace('w','W'));
//数组名.replace('被替换元素','替换元素');
one2=one2.replace('e','E');
System.out.println(one2);
System.out.println(one3.replace("world!", "Java!"));
}
}
判断两数组是否相等使用equals()方法与equalsIgnoreCase()方法,其返回值为boolean值。判断两数组是否相等直接使用”==“运算符是错误的。equals()方法区分大小写,则不区分大小写。
public class StringDemo{
public static void main(String[] args){
String one1,one2,one3;
one1="Hello,wrold!";
one2="Hello,Wrold!";
System.out.println(one1.equals(one2));
System.out.println(one1.equalsIgnoreCase(one2));
}
}
字母大小写转换使用toLowerCase()方法与toUpperCase()方法。toLowerCase()方法是将字符串中的大小字母转换成小写字母,toUpperCase()方法则是将字符串中的小写字母转换成大小写字母。
public class StringDemo{
public static void main(String[] args){
String one="Hello,world!";
System.out.println(one.toLowerCase());
System.out.println(one.toUpperCase());
}
}
关于字符串的基本操作就讲解到这里,如果需要进行深入学习的同学可以去研究下Java的API文档,这里附上在线Java API文档地址http://tool.oschina.net/apidocs/apidoc?api=jdk-zh在浏览器中可以使用搜索进行查找,快捷键为Ctrl+F。
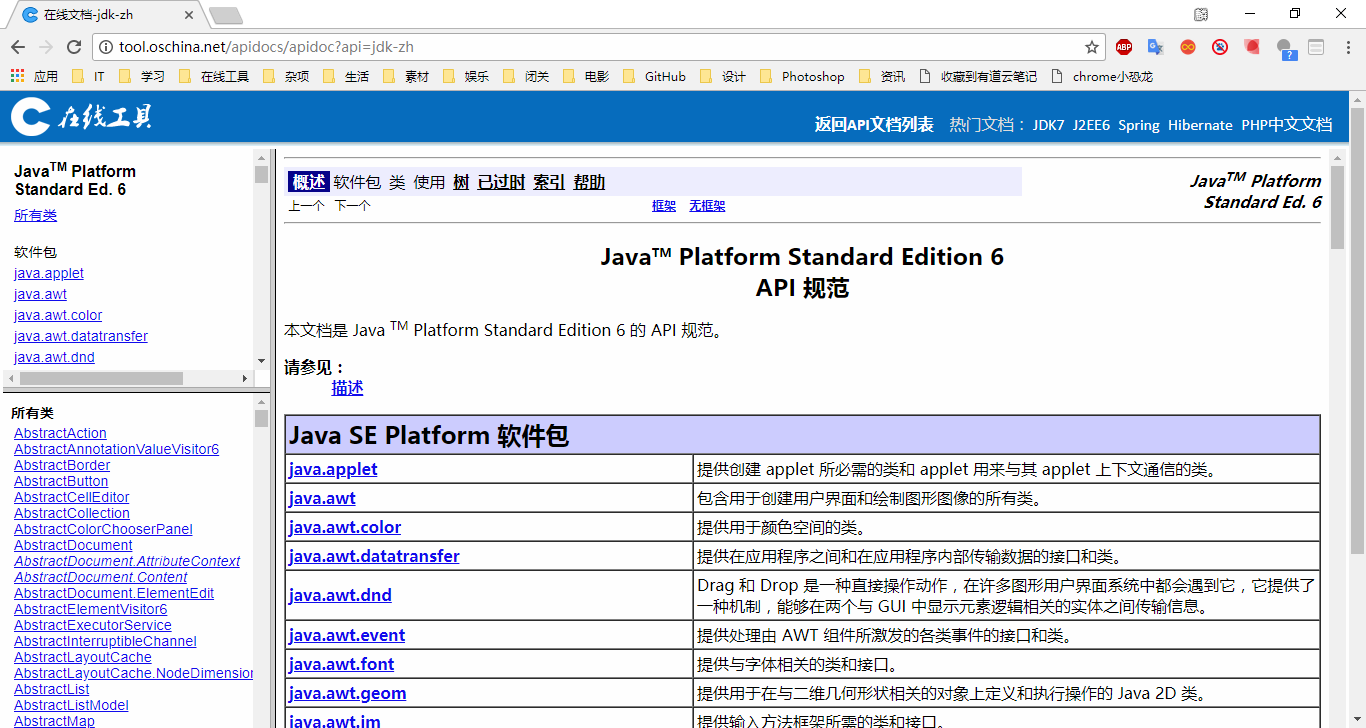
在Java中字符串被当作对象处理,使用String类来创建、操作字符串对象。String类在lang包中,这里我们不需要进行导包。(lang包是Java的核心包,JVM默认导入而不需要我们再进行显示导入)
这里讲解一下Java中最常用的两种创建数组的方法,方法1使用字符串常量来创建字符串变量。方法2则是使用字符串数组来创建字符串变量。使用字符数组来创建字符串变量还可以将数组中一部分元素提取出来创建字符串变量。具体实现见例程中方法2-2,其操作的元素也是只包括起始范围的值,不包括结束范围的值。字符串必须包含子啊双引号里,声明过后必须初始化才能使用。
public class StringDemo{
public static void main(String[] args){
String one;
one="Hello";
System.out.println(one);//方法1
char two1[]= {'h','e','l','l','o'};
String three1=new String(two1);
System.out.println(three1);//方法2-1
char two2[]= {'h','e','l','l','o'};
String three2=new String(two2,0,2);
System.out.println(three2);//方法2-2
}
}
了解了字符串的声明与创建后我们来学习一下字符串的基本操作,连接、获取字符串长度、查找、获取指定索引位置字符、获取子字符串、去除空格、分割、替换……两个字符串使用“+”运算符可以进行连接,拼合成一个新的字符串。Java中一个字符串是不能分开两行写的,如果字符串过长要分两行写的话也需要使用“+”运算符进行连接,否则会报错。
public class StringDemo{
public static void main(String[] args){
String one,two,three,four;
one="Hello,";
two="world!";
three=one+""+two;
System.out.println(one);
System.out.println(two);
System.out.println(three);
four="Java is "+
"gret";
System.out.println(four);
System.out.println("Java is "+
"gret!");
}
}
除了字符串之间相连接外,还可以与其它基本数据类型进行连接(其它数据类型数据会被转换成字符串,“+”运算符只要有一个操作数是字符串另一个操作数就会被编译器转换成字符串)。
public class StringDemo{
public static void main(String[] args){
int one=2;
float two=2.2F;
String three="版本:";
three=three+one;
System.out.println(three);
System.out.println(three+one+two);
System.out.println(three+(one+two));
}
}
获取字符串长度使用length()方法
public class StringDemo{
public static void main(String[] args){
String one="Hello,world!";
String two="Hello,java!";
int size=one.length();
System.out.println(size);
System.out.println(two.length());
}
}
获取指定索引位置字符使用charAt()方法
public class StringDemo{
public static void main(String[] args){
String one="Hello,world!";
char two=one.charAt(2);
System.out.println(two);
System.out.println(one.charAt(6));
}
}
字符串查找String类提供了indexOf()、lastIndexOf()两种方法。indexOf返回的是被搜素字符(字符串)首次出现位置,lastIndexOf()则是返回被搜素字符(字符串)最后一次出现位置。若没有检索到则会返回-1。
public class StringDemo{
public static void main(String[] args){
String one="Hello,world!";
System.out.println(one.indexOf("w"));
System.out.println(one.indexOf("a"));
System.out.println(one.lastIndexOf("l"));
System.out.println(one.lastIndexOf("a"));
}
}
获取子字符串使用substring()方法,可利用下标对字符串进行截取操作。有两种不同的方法重载。
public class StringDemo{
public static void main(String[] args){
String one="Hello,world!";
System.out.println(one.substring(2));//方法1-从指定下标处开始截取
System.out.println(one.substring(2,6));//方法2-截取指定范围内元素
}
}
去除空格使用trim()方法。trim()方法去除的是首尾空格,这种空格一般无意义而且会影响字符串操作、占用空间。
public class StringDemo{
public static void main(String[] args){
String one=" Hello world ";
String two;
System.out.println(one.length());
System.out.println(one);
System.out.println(one.trim().length());
two=one.trim();
System.out.println(two);
System.out.println(two.length());
}
}
字符串替换使用replace()方法。
public class StringDemo{
public static void main(String[] args){
String one1="Hello,world!";
String one2="Hello,world!";
String one3="Hello,world!";
System.out.println(one1.replace('w','W'));
//数组名.replace('被替换元素','替换元素');
one2=one2.replace('e','E');
System.out.println(one2);
System.out.println(one3.replace("world!", "Java!"));
}
}
判断两数组是否相等使用equals()方法与equalsIgnoreCase()方法,其返回值为boolean值。判断两数组是否相等直接使用”==“运算符是错误的。equals()方法区分大小写,则不区分大小写。
public class StringDemo{
public static void main(String[] args){
String one1,one2,one3;
one1="Hello,wrold!";
one2="Hello,Wrold!";
System.out.println(one1.equals(one2));
System.out.println(one1.equalsIgnoreCase(one2));
}
}
字母大小写转换使用toLowerCase()方法与toUpperCase()方法。toLowerCase()方法是将字符串中的大小字母转换成小写字母,toUpperCase()方法则是将字符串中的小写字母转换成大小写字母。
public class StringDemo{
public static void main(String[] args){
String one="Hello,world!";
System.out.println(one.toLowerCase());
System.out.println(one.toUpperCase());
}
}
关于字符串的基本操作就讲解到这里,如果需要进行深入学习的同学可以去研究下Java的API文档,这里附上在线Java API文档地址http://tool.oschina.net/apidocs/apidoc?api=jdk-zh在浏览器中可以使用搜索进行查找,快捷键为Ctrl+F。
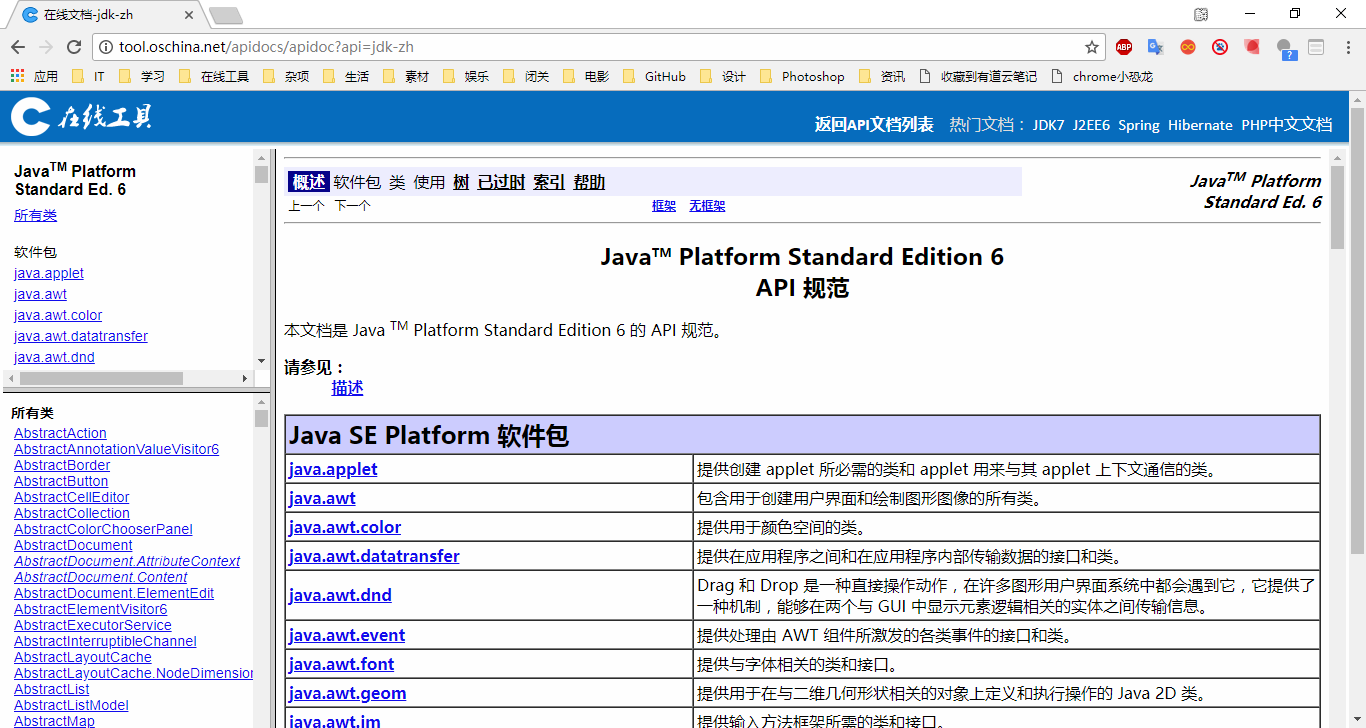
本文由IT教学网整理发布,转载请注明出处:http://www.itjx.com/jiaocheng/java/455.html